Understanding the Request-Response Cycle in Node.js
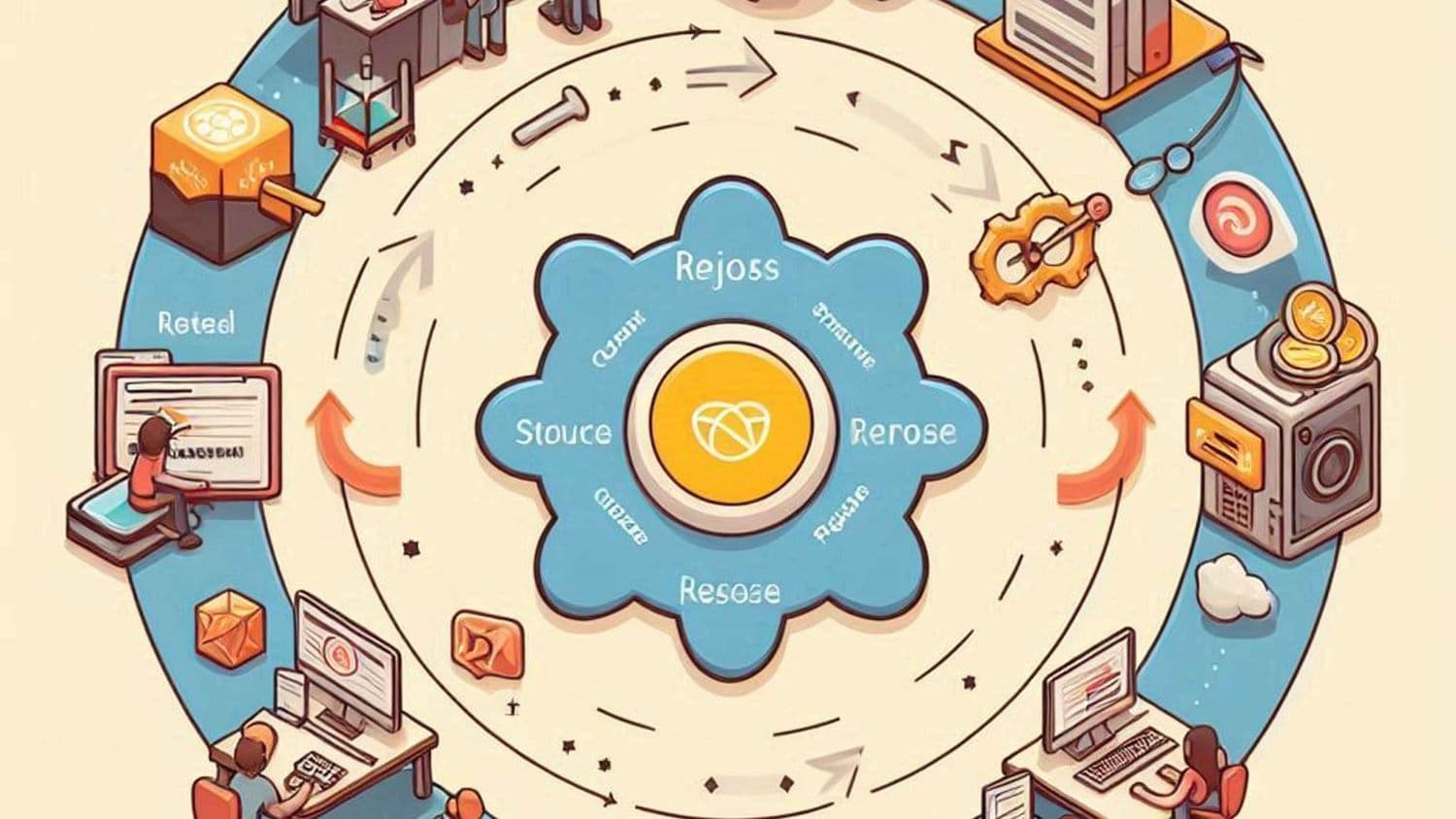
Node.js has revolutionized the way we build web applications by providing a non-blocking, event-driven architecture. At the core of every web application is the request-response cycle, a fundamental concept that dictates how servers interact with clients. In this blog post, we'll explore how the request-response cycle works in Node.js, breaking it down into easy-to-understand components.
What is the Request-Response Cycle?
The request-response cycle is the process by which a client (usually a web browser) sends a request to a server, which then processes that request and returns a response. This cycle is essential for enabling communication over the web.
1. Client Sends a Request
The cycle begins when a client sends a request to the server. This can happen in various ways, such as when a user enters a URL in their browser, clicks a link, or submits a form. The request includes:
- HTTP Method: Such as GET, POST, PUT, DELETE, etc., indicating what action the client wants to perform.
- URL: The endpoint being requested.
- Headers: Metadata about the request, such as content type, authentication tokens, etc.
- Body (optional): Data sent with the request, mainly for POST and PUT methods.
2. Server Receives the Request
When the server receives the request, it typically goes through several layers of processing. In a Node.js application, this is often handled using the Express framework, which simplifies routing and middleware management. Here’s a brief overview of the server-side processing:
- Routing: The server checks the request’s URL and HTTP method to determine which route handler should process it.
- Middleware: Node.js applications frequently use middleware functions to perform operations such as logging, authentication, parsing request bodies, and error handling before reaching the final route handler.
3. Processing the Request
Once the appropriate route handler is identified, it processes the request. This may involve various actions:
- Database Queries: The handler might need to interact with a database to fetch or store data.
- Business Logic: Implementing any application-specific logic to prepare the data or response.
- Formatting the Response: The server prepares the response in the required format (usually JSON or HTML).
4. Server Sends a Response
After processing the request, the server generates a response, which includes:
- HTTP Status Code: Indicates whether the request was successful (e.g., 200 for success, 404 for not found, 500 for server error).
- Headers: Similar to the request, these provide metadata about the response.
- Body: The actual content being sent back to the client, such as HTML, JSON, or plain text.
5. Client Receives the Response
Finally, the client receives the response. The web browser or client application processes this response, which may involve rendering a new page, updating existing content, or displaying error messages based on the status code.
Example of a Simple Request-Response Cycle in Node.js
Here’s a simple example using Express to illustrate the request-response cycle:
const express = require('express');
const app = express();
// Middleware to parse JSON request bodies
app.use(express.json());
// Route handler for a GET request
app.get('/api/data', (req, res) => {
// Simulate fetching data
const data = { message: 'Hello, World!' };
res.status(200).json(data);
});
// Route handler for a POST request
app.post('/api/data', (req, res) => {
const newData = req.body; // Get data from request body
// Simulate saving data (in a real app, you would save this to a database)
res.status(201).json({ message: 'Data received', data: newData });
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Explanation of the Example
- Setting Up Express: We create an Express application.
- Middleware: We use express.json() to parse incoming JSON request bodies.
- GET Request: When the client sends a GET request to /api/data, the server responds with a JSON object.
- POST Request: When the client sends a POST request to the same endpoint, the server responds with a confirmation message along with the received data.
- Server Listening: The server listens on a specified port, waiting for incoming requests.
Conclusion
The request-response cycle is a fundamental aspect of web development, and understanding it is crucial for building efficient Node.js applications. By grasping how requests are processed and responses are generated, developers can create more robust, scalable, and responsive applications.
As you dive deeper into Node.js, keep experimenting with different aspects of the request-response cycle, such as error handling, middleware, and asynchronous operations. Happy coding! 👍