Mastering API Requests in JavaScript: A Comprehensive Guide
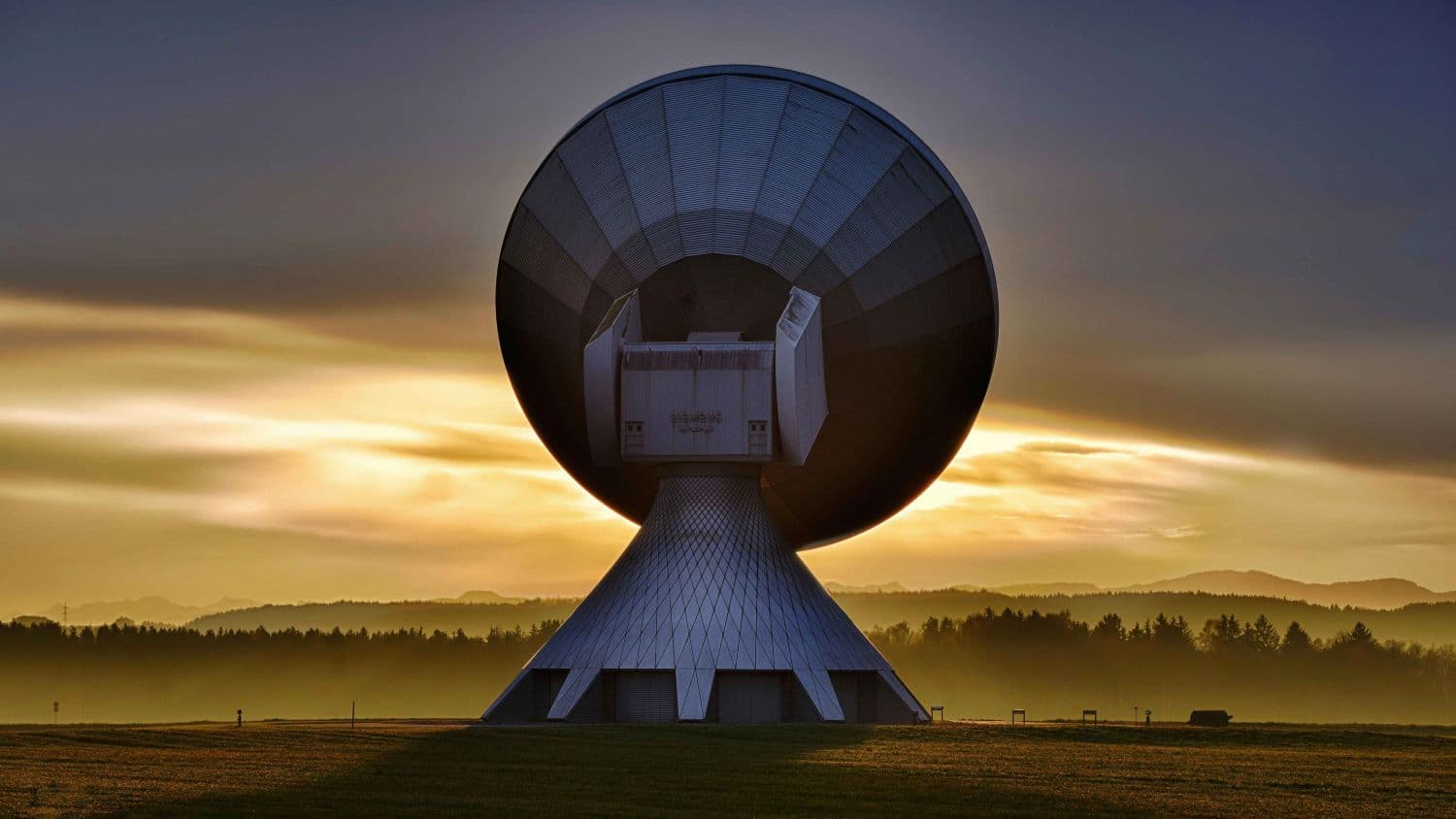
APIs (Application Programming Interfaces) are essential for building modern web applications, allowing them to communicate with external services and retrieve or send data. JavaScript, with its versatility and popularity, provides several methods to make API requests. In this blog, we’ll explore various ways to make API requests in JavaScript, covering fetch, XMLHttpRequest, and libraries like Axios.
1. Using Fetch API
The Fetch API is a modern interface for making network requests. It’s promise-based, making it easier to handle asynchronous operations. Here's how to use it for different types of requests.
GET Request
A GET request retrieves data from the server. Here’s how you can perform a GET request using the Fetch API:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('There has been a problem with your fetch operation:', error));
POST Request
A POST request sends data to the server. Here’s how to perform it:
fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ key: 'value' }),
})
.then(response => response.json())
.then(data => console.log('Success:', data))
.catch(error => console.error('Error:', error));
PUT Request
A PUT request updates existing data on the server. Here’s an example:
fetch('https://api.example.com/data/1', {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ key: 'newValue' }),
})
.then(response => response.json())
.then(data => console.log('Updated:', data))
.catch(error => console.error('Error:', error));
DELETE Request
To delete data from the server, you can use a DELETE request:
fetch('https://api.example.com/data/1', {
method: 'DELETE',
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
console.log('Deleted successfully');
})
.catch(error => console.error('Error:', error));
2. Using XMLHttpRequest
Though it’s less common now due to the Fetch API's simplicity, XMLHttpRequest is still widely used. Here’s how to make different types of requests with it.
GET Request
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(JSON.parse(xhr.responseText));
} else {
console.error('Request failed:', xhr.statusText);
}
};
xhr.onerror = function () {
console.error('Request failed');
};
xhr.send();
POST Request
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.example.com/data', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log('Success:', JSON.parse(xhr.responseText));
} else {
console.error('Request failed:', xhr.statusText);
}
};
xhr.send(JSON.stringify({ key: 'value' }));
PUT Request
const xhr = new XMLHttpRequest();
xhr.open('PUT', 'https://api.example.com/data/1', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log('Updated:', JSON.parse(xhr.responseText));
} else {
console.error('Request failed:', xhr.statusText);
}
};
xhr.send(JSON.stringify({ key: 'newValue' }));
DELETE Request
const xhr = new XMLHttpRequest();
xhr.open('DELETE', 'https://api.example.com/data/1', true);
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log('Deleted successfully');
} else {
console.error('Request failed:', xhr.statusText);
}
};
xhr.send();
3. Using Axios
Axios is a promise-based HTTP client that simplifies the process of making API requests. It supports older browsers, offers a rich feature set, and has a simple syntax. First, install Axios via npm or include it in your project:
npm install axios
GET Request
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
POST Request
axios.post('https://api.example.com/data', { key: 'value' })
.then(response => console.log('Success:', response.data))
.catch(error => console.error('Error:', error));
PUT Request
axios.put('https://api.example.com/data/1', { key: 'newValue' })
.then(response => console.log('Updated:', response.data))
.catch(error => console.error('Error:', error));
DELETE Request
axios.delete('https://api.example.com/data/1')
.then(response => console.log('Deleted successfully'))
.catch(error => console.error('Error:', error));
Conclusion
Making API requests in JavaScript is straightforward, whether you choose to use the Fetch API, XMLHttpRequest, or a library like Axios. Each method has its pros and cons, but Fetch and Axios are preferred for their simplicity and modern capabilities. Understanding these techniques will empower you to interact with APIs effectively, opening up a world of possibilities for your web applications.
Happy coding! 👍